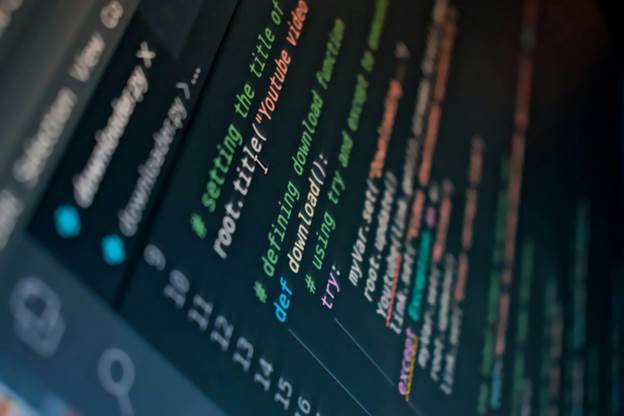
Because good code isn’t just about making it work, it’s about making it easy to understand.
Let’s be honest, learning to code can feel like learning a new language. (Because, well, it is.) And just like with speaking or writing, how clearly you communicate matters. That’s why writing clean, readable code is one of the most important skills every programmer should learn, especially if you’re just starting out.
Use Meaningful Variable Names
Ever seen variables named a, b, or temp? While quick to type, they leave you scratching your head later. Instead:
- Be descriptive: Use totalScore instead of ts1.
- Keep it concise: userAge is better than theAgeOfTheUser.
- Stick to a style: camelCase (userScore) or snake_case (user_score) but pick one and be consistent.
Good names act like signposts, guiding readers through your code. They tell the story of what each piece does without needing extra comments.
For Example, If you are calculating the area, instead of this:
x = 10
y = 5
z = x * y
Write this:
length = 10
width = 5
area = length * width
Break Code into Small, Focused Functions
Imagine your teacher giving you one giant task instead of several steps. Overwhelming! In coding:
- One job per function: A function named calculateAverage() should just calculate averages, not print results or read files.
- Short and sweet: Aim for functions no longer than 20–30 lines.
- Reuse whenever possible: If you see the same pattern in multiple places, pull it into its own function.
Small functions are easier to test, debug, and understand. When something goes wrong, you know exactly where to look.
Also, break your code into sections using:
- Blank lines
- Comments
- Functions
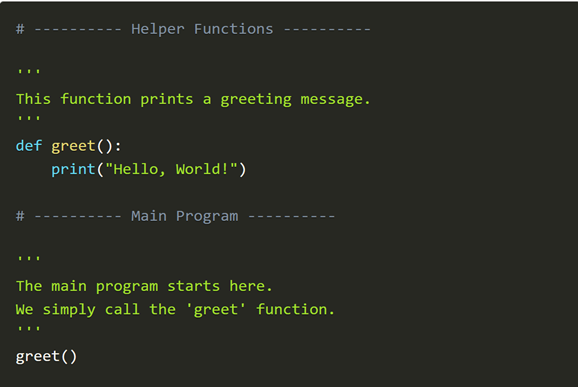
Comment Smartly – Not Everywhere
Comments can be lifesavers, but over-commenting turns your code into a cluttered mess. Instead:
- Explain “why,” not “what”: If you write // increment i by 1, that’s obvious. But // using +1 here to align with user index starting at 1 adds valuable context.
- Keep comments up to date: Stale comments are more confusing than no comments.
- Providing comprehensive comments within the code helps other developers comprehend the code’s purpose or functionality, fostering better collaboration and communication within the team.
The code example below shows how you can use multiline comments to enhance collaboration:
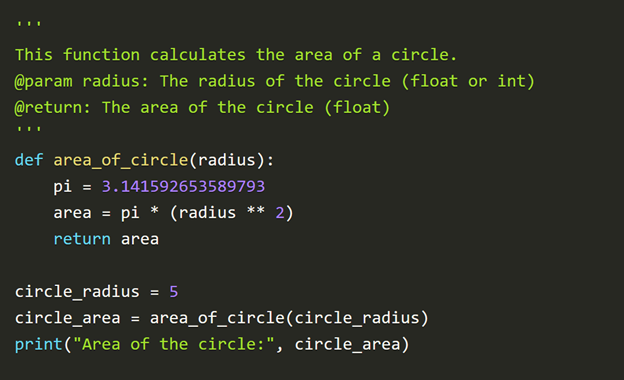
Test Often and Fix Early
Rather than waiting until the very end to see if everything works, add testing into your coding routine. By testing small pieces of your code as you write them, you catch bugs early when they’re easiest to understand and fix rather than hunting through hundreds of lines later on. This approach saves time, reduces frustration, and helps you gain confidence that each part of your project works correctly before moving on.
Example 1: Quick Unit Test for a Helper Function
After writing a function to calculate the average of a list of numbers, you can add a tiny test right below it to verify it works as expected:
def calculate_average(scores):
return sum(scores) / len(scores)
# Quick test: if this fails, you know to fix calculate_average before using it elsewhere
assert calculate_average([10, 20, 30]) == 20
Here, running your script will immediately alert you if calculate_average ever returns the wrong result so you can correct it before it sneaks into larger parts of your program.
Example 2: Playtest Your Game After Each Feature
If you’re building a simple text adventure or game, don’t wait until the end to try it out. After adding a new room, puzzle, or command, run through your game to make sure that nothing else broke:
- Add the “pick up key” command.
- Restart the game and type “pick up key.”
- Verify your inventory shows the key.
By playtesting each addition immediately, you’ll notice issues like the key not appearing or the command misfiring right away, making them much easier to track down and resolve.
- Use Version Control
Think of version control like the “save game” feature in your favorite video game or the revision history in Google Docs it keeps track of every change you make so you can go back if something breaks. For programmers, the most popular tool is Git, and services like GitHub let you store your code in the cloud and collaborate with others.
Why it helps,
- Track your progress: Every “save” (called a commit) records exactly what changed.
- Undo mistakes: If you introduce a bug, you can roll back to an earlier commit.
- Collaborate easily: Multiple people can work on the same project without overwriting each other’s work.
Example
- Start tracking your project folder:
cd my-cool-project
git init
- Save your first version:
git add.
git commit -m “Initial commit: added main.py with calculate_average function”
- Make a change, then save again:
# Edit main.py to add a new helper function…
git add main.py
git commit -m “Add helper function to format output”
Now, if the new helper function causes an issue, you can run git log to see all your commits, then use git checkout to go back to any earlier version. This safety net means you can experiment without fear—if something goes wrong, just rewind!
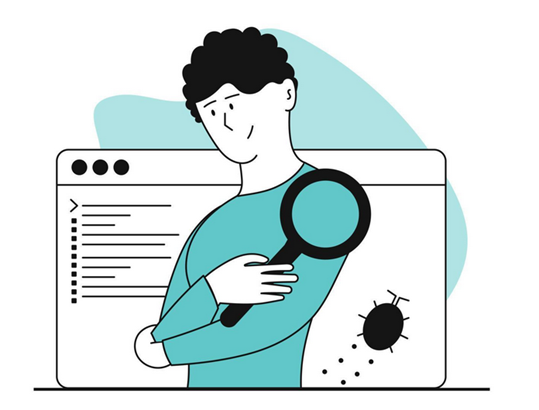